Add a Layer to a Map with an Activity
Some activities may need access to resources like the map, the host application or host widget. The AppActivity
base class can be used to extend an existing activity to access these resources.
This article will walk you through creating a custom activity that adds a new layer to the map.
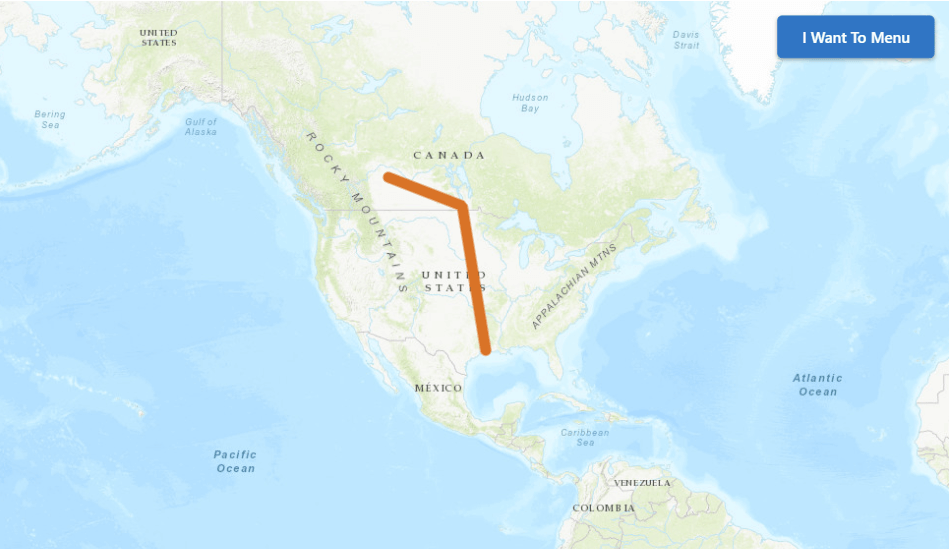
Prerequisites
Follow the instructions in the Web Applications SDK page to set up your development environment.
note
A working knowledge of TypeScript is recommended before extending Workflow for web-based hosts.
Setup the Activity
- Open up a terminal shell in the SDK folder.
- Run the command
npm run activity
and create a new activityAddLayerToMap
.
Modify the Activity to Access App Properties
- Add an import for the
AppActivity
base class at the top of theAddLayerToMap.ts
file.import { AppActivity } from "@geocortex/workflow/runtime/app/AppActivity";
- Modify the activity class declaration to extend the
AppActivity
base class.export class AddLayerToMap extends AppActivity
Add a New Map Layer with the Activity
Next, we can use the ArcGIS Runtime SDK for .NET and the AppActivity
class to create a graphics layer and add it to the host's map through the this.map
property.
note
This activity assumes that it will be running in Geocortex Web, and so it uses the 4.x ArcGIS API for Javascript.
Deploy the Activity
Follow the instructions to build the activity pack and deploy it.
Test the Activity
Once your activity pack is hosted and registered, your custom activity should appear in the activity toolbox in Geocortex Workflow Designer alongside the built-in activities, and can be used in the graphical interface like any other activity.
Now you can build a workflow that uses your new custom activity!
note
You can download this demo workflow that uses the custom activity and then import it into the Geocortex Workflow Designer. You will have to deploy the custom activity and form element for it to function. This workflow assumes you are hosting the activity pack with the dev server on https://localhost:57999/.
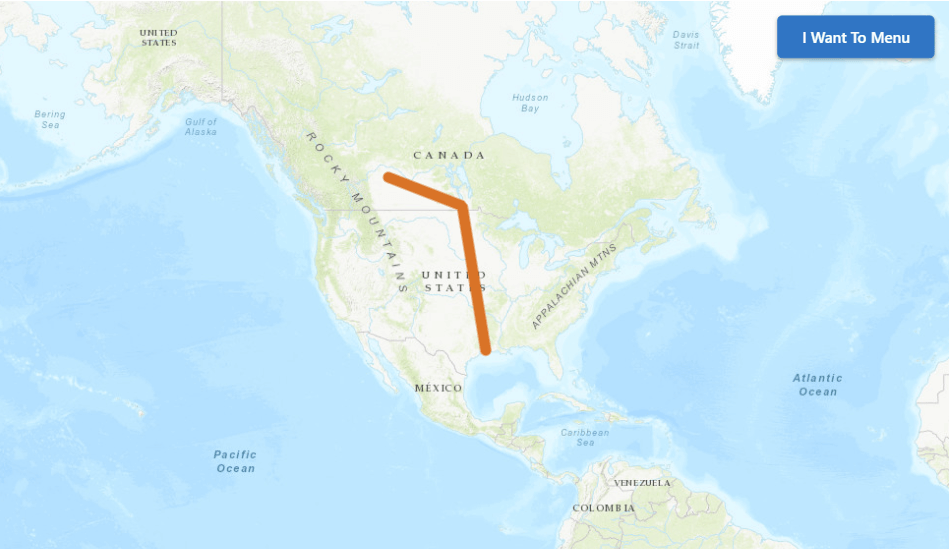